mod_time Module Reference
Data Types | |
interface | abs |
interface | assignment(=) |
interface | days2time |
interface | mod |
interface | operator(*) |
interface | operator(+) |
interface | operator(-) |
interface | operator(/) |
interface | operator(/=) |
interface | operator(<) |
interface | operator(<=) |
interface | operator(==) |
interface | operator(>) |
interface | operator(>=) |
interface | seconds2time |
type | time |
Functions/Subroutines | |
type(time) function | abs_time (A) |
type(time) function | modulo_time (A, B) |
type(time) function | days2time_dbl (DAYS) |
type(time) function | days2time_int (DAYS) |
type(time) function | days2time_lint (DAYS) |
type(time) function | days2time_flt (DAYS) |
type(time) function | seconds2time_dbl (SECS) |
type(time) function | seconds2time_int (SECS) |
type(time) function | seconds2time_lint (SECS) |
type(time) function | seconds2time_flt (SECS) |
integer function | time2ncitime (MJD, RJD, D, MS) |
type(time) function | ncitime (D, MS) |
subroutine | adjust (MJD) |
type(time) function | read_time (timestr, status, TZONE) |
type(time) function | time_zone (TZONE, status) |
logical function | is_valid_timezone (timezone) |
type(time) function | read_datetime (timestr, frmt, TZONE, status) |
character(len=80) function | write_datetime (mjdin, prec, TZONE) |
type(time) function | get_now () |
real(dp) function | seconds (MJD) |
real(dp) function | days (MJD) |
type(time) function | int_x_time (int, MJD) |
type(time) function | long_x_time (long, MJD) |
type(time) function | time_x_int (MJD, int) |
type(time) function | time_x_long (MJD, long) |
type(time) function | time_x_flt (MJD, flt) |
type(time) recursive function | time_x_dbl (MJD, dbl) |
type(time) function | flt_x_time (flt, MJD) |
type(time) function | dbl_x_time (dbl, MJD) |
type(time) function | time_div_int (MJD, int) |
type(time) function | time_div_long (MJD, long) |
type(time) function | time_div_flt (MJD, flt) |
type(time) function | time_div_dbl (MJD, dbl) |
type(time) function | add_time (time1, time2) |
type(time) function, dimension(size(time1)) | add_time_1 (time1, time2) |
type(time) function, dimension(size(time1)) | add_time_1a (time1, time2) |
type(time) function, dimension(size(time2)) | add_time_a1 (time1, time2) |
type(time) function, dimension(size(time1, 1), size(time1, 2)) | add_time_2 (time1, time2) |
type(time) function, dimension(size(time1, 1), size(time1, 2)) | add_time_2a (time1, time2) |
type(time) function, dimension(size(time2, 1), size(time2, 2)) | add_time_a2 (time1, time2) |
type(time) function | subtract_time (time1, time2) |
type(time) function, dimension(size(time1)) | subtract_time_1 (time1, time2) |
type(time) function, dimension(size(time2)) | subtract_time_1a (time1, time2) |
type(time) function, dimension(size(time1)) | subtract_time_a1 (time1, time2) |
type(time) function, dimension(size(time1, 1), size(time1, 2)) | subtract_time_2 (time1, time2) |
type(time) function, dimension(size(time1, 1), size(time1, 2)) | subtract_time_2a (time1, time2) |
type(time) function, dimension(size(time2, 1), size(time2, 2)) | subtract_time_a2 (time1, time2) |
subroutine | assign_time (A, B) |
logical function | le_time (time1, time2) |
logical function | lt_time (time1, time2) |
logical function | eq_time (time1, time2) |
logical function | ne_time (time1, time2) |
logical function | ge_time (time1, time2) |
logical function | gt_time (time1, time2) |
subroutine | print_time (mjd, IPT, char) |
subroutine | print_real_time (mjd, IPT, char, TZONE) |
subroutine | now_2_month_days (TTime, Pyear, Pmonth, Pmdays) |
subroutine | now_2_days_test |
Variables | |
integer, parameter | itime = SELECTED_INT_KIND(18) |
integer | mpi_time |
integer(itime), parameter | spd = 86400 |
integer(itime), parameter | mspd = spd * 1000 |
integer(itime), parameter | muspd = mspd * 1000 |
integer(itime), parameter | million = 10**6 |
Function/Subroutine Documentation
Here is the call graph for this function:

type(time) function mod_time::add_time | ( | type(time), intent(in) | time1, |
type(time), intent(in) | time2 | ||
) |
Here is the call graph for this function:

type(time) function, dimension(size(time1)) mod_time::add_time_1 | ( | type(time), dimension(:), intent(in) | time1, |
type(time), dimension(:), intent(in) | time2 | ||
) |
type(time) function, dimension(size(time1)) mod_time::add_time_1a | ( | type(time), dimension(:), intent(in) | time1, |
type(time), intent(in) | time2 | ||
) |
type(time) function, dimension(size(time1,1),size(time1,2)) mod_time::add_time_2 | ( | type(time), dimension(:,:), intent(in) | time1, |
type(time), dimension(:,:), intent(in) | time2 | ||
) |
type(time) function, dimension(size(time1,1),size(time1,2)) mod_time::add_time_2a | ( | type(time), dimension(:,:), intent(in) | time1, |
type(time), intent(in) | time2 | ||
) |
type(time) function, dimension(size(time2)) mod_time::add_time_a1 | ( | type(time), intent(in) | time1, |
type(time), dimension(:), intent(in) | time2 | ||
) |
type(time) function, dimension(size(time2,1),size(time2,2)) mod_time::add_time_a2 | ( | type(time), intent(in) | time1, |
type(time), dimension(:,:), intent(in) | time2 | ||
) |
subroutine mod_time::adjust | ( | type(time) | MJD | ) |
Here is the caller graph for this function:
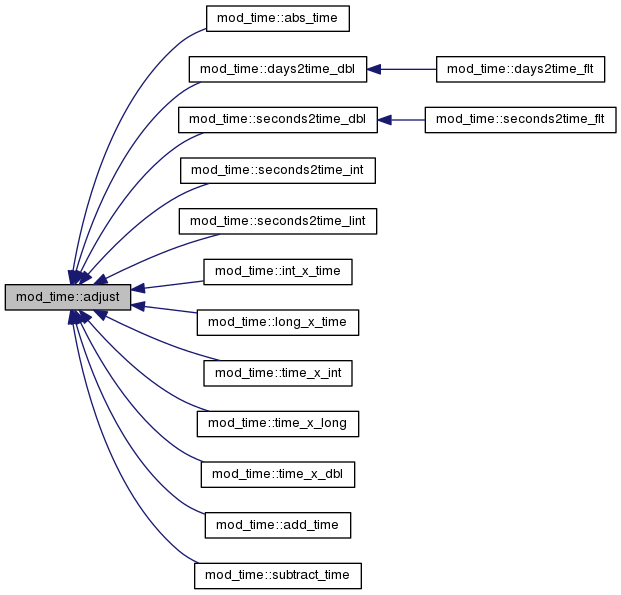
real(dp) function mod_time::days | ( | type(time), intent(in) | MJD | ) |
Here is the caller graph for this function:
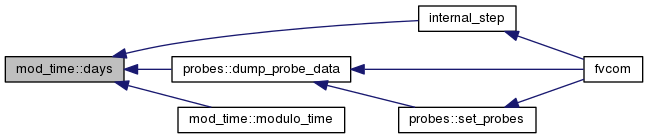
type(time) function mod_time::days2time_dbl | ( | real(dp), intent(in) | DAYS | ) |
Here is the call graph for this function:

Here is the caller graph for this function:

type(time) function mod_time::days2time_flt | ( | real(spa), intent(in) | DAYS | ) |
Here is the call graph for this function:

type(time) function mod_time::days2time_int | ( | integer, intent(in) | DAYS | ) |
Here is the caller graph for this function:

type(time) function mod_time::get_now | ( | ) |
Here is the call graph for this function:

Here is the caller graph for this function:
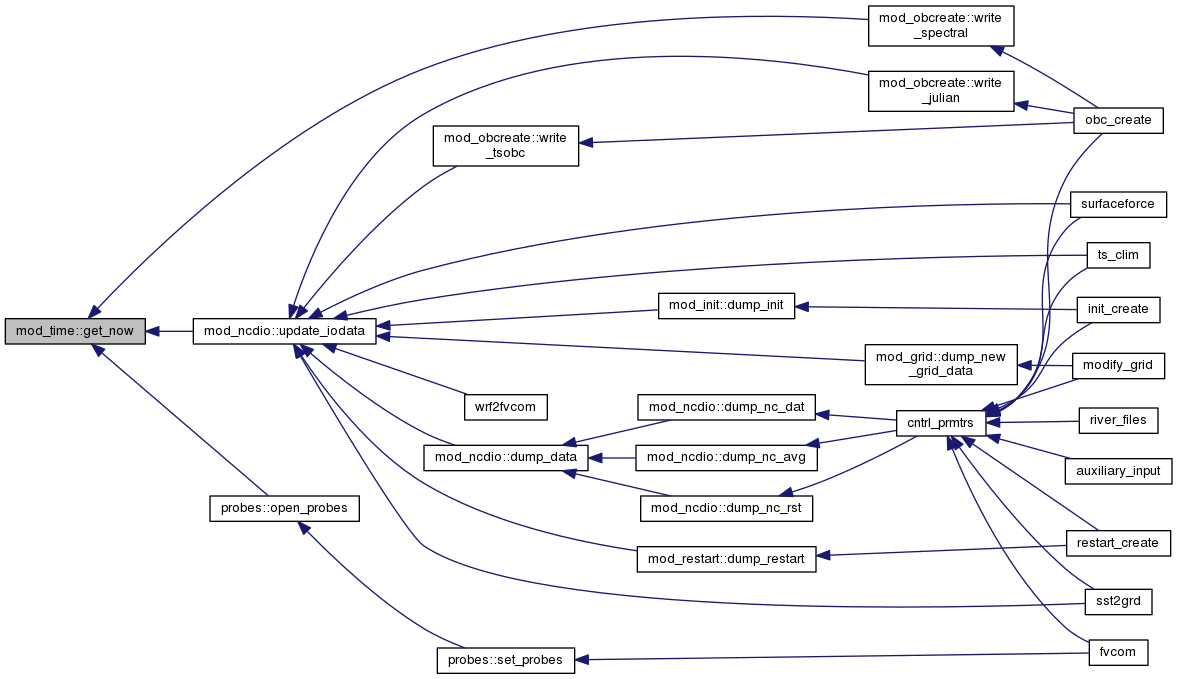
Here is the call graph for this function:

logical function mod_time::is_valid_timezone | ( | character(len=*), intent(in) | timezone | ) |
Here is the call graph for this function:

Here is the caller graph for this function:
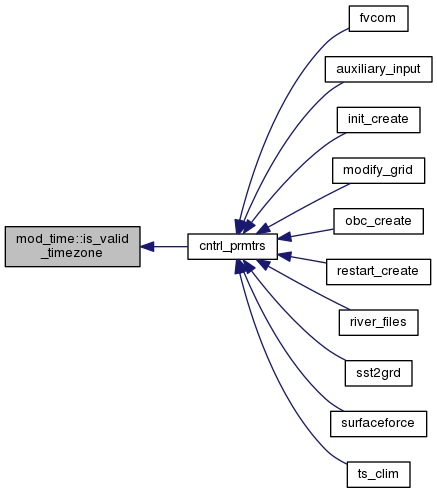
type(time) function mod_time::long_x_time | ( | integer(itime), intent(in) | long, |
type(time), intent(in) | MJD | ||
) |
Here is the call graph for this function:

Here is the call graph for this function:

type(time) function mod_time::ncitime | ( | integer, intent(in) | D, |
integer, intent(in) | MS | ||
) |
Here is the call graph for this function:

subroutine mod_time::now_2_days_test | ( | ) |
Here is the call graph for this function:

subroutine mod_time::now_2_month_days | ( | type(time), intent(in) | TTime, |
integer, intent(out) | Pyear, | ||
integer, intent(out) | Pmonth, | ||
integer, intent(out) | Pmdays | ||
) |
Here is the call graph for this function:

Here is the caller graph for this function:
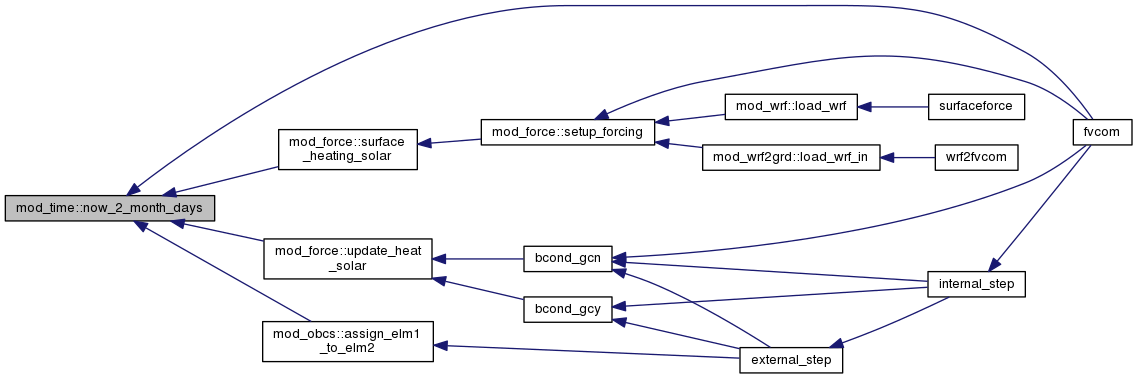
subroutine mod_time::print_real_time | ( | type(time), intent(in) | mjd, |
integer, intent(in) | IPT, | ||
character(len=*), intent(in) | char, | ||
character(len=*), intent(in), optional | TZONE | ||
) |
Here is the call graph for this function:

Here is the caller graph for this function:
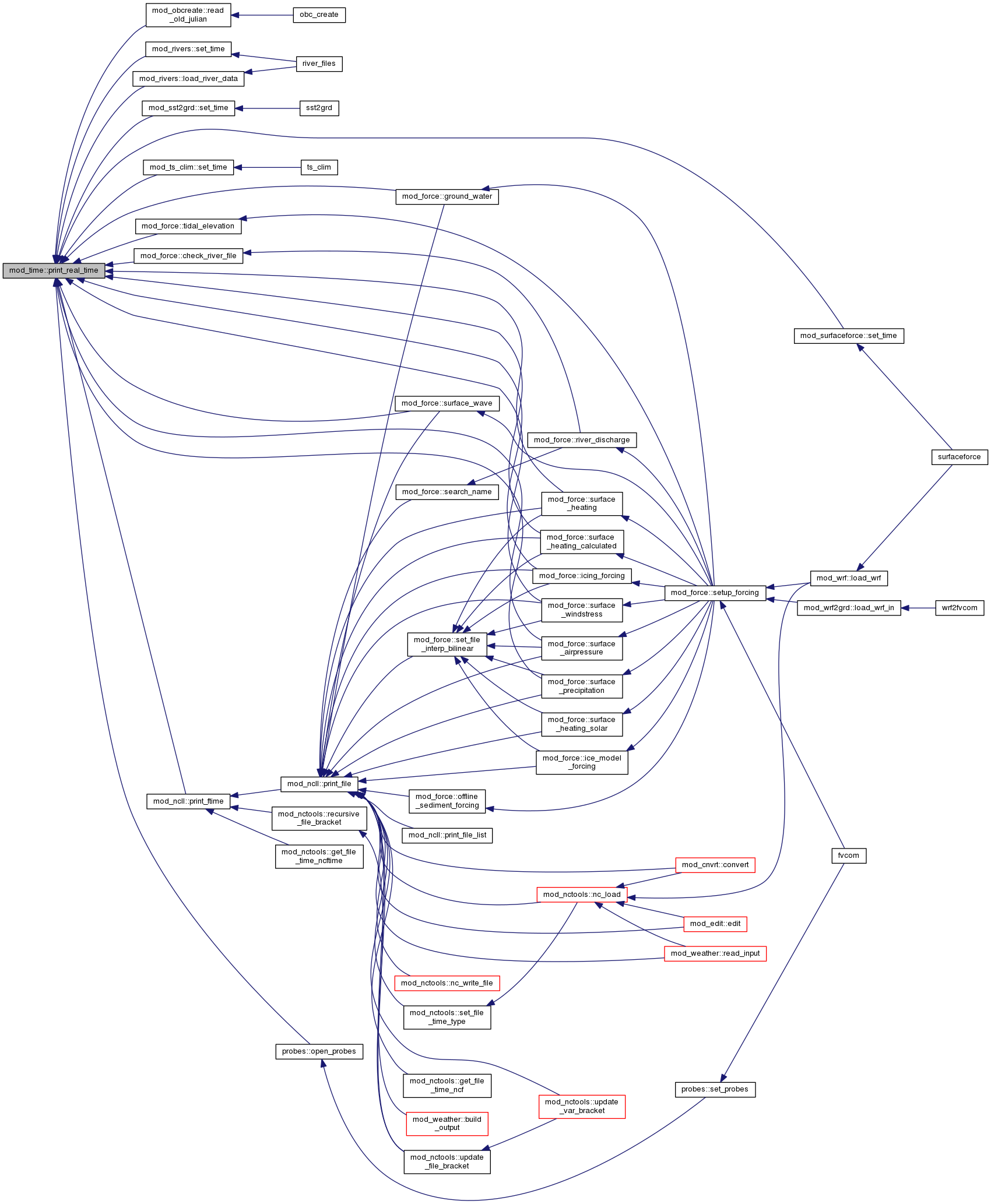
subroutine mod_time::print_time | ( | type(time), intent(in) | mjd, |
integer, intent(in) | IPT, | ||
character(len=*), intent(in) | char | ||
) |
Here is the caller graph for this function:
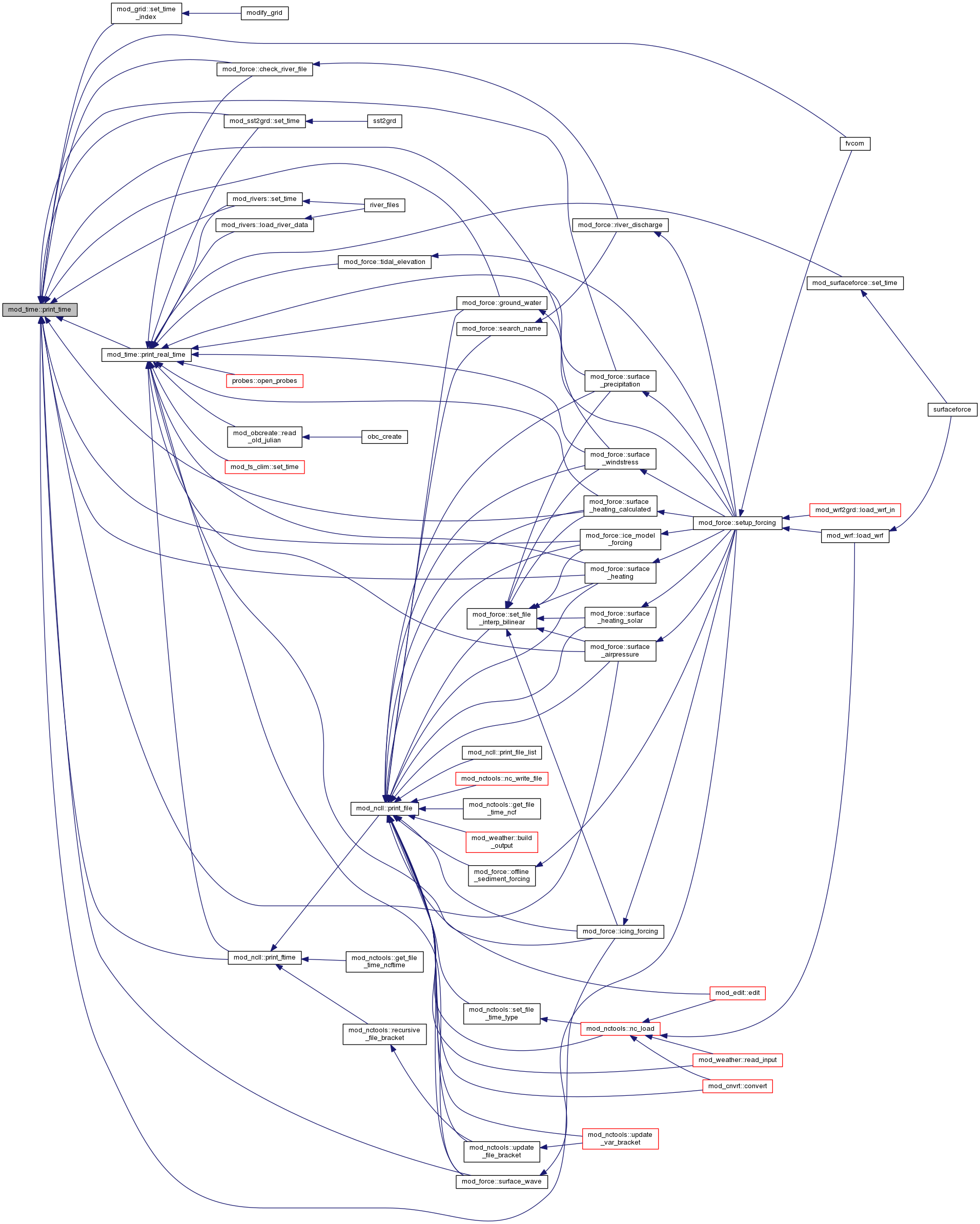
type(time) function mod_time::read_datetime | ( | character(len=*) | timestr, |
character(len=*), intent(in) | frmt, | ||
character(len=*), intent(in) | TZONE, | ||
integer, intent(out) | status | ||
) |
Here is the call graph for this function:

Here is the caller graph for this function:
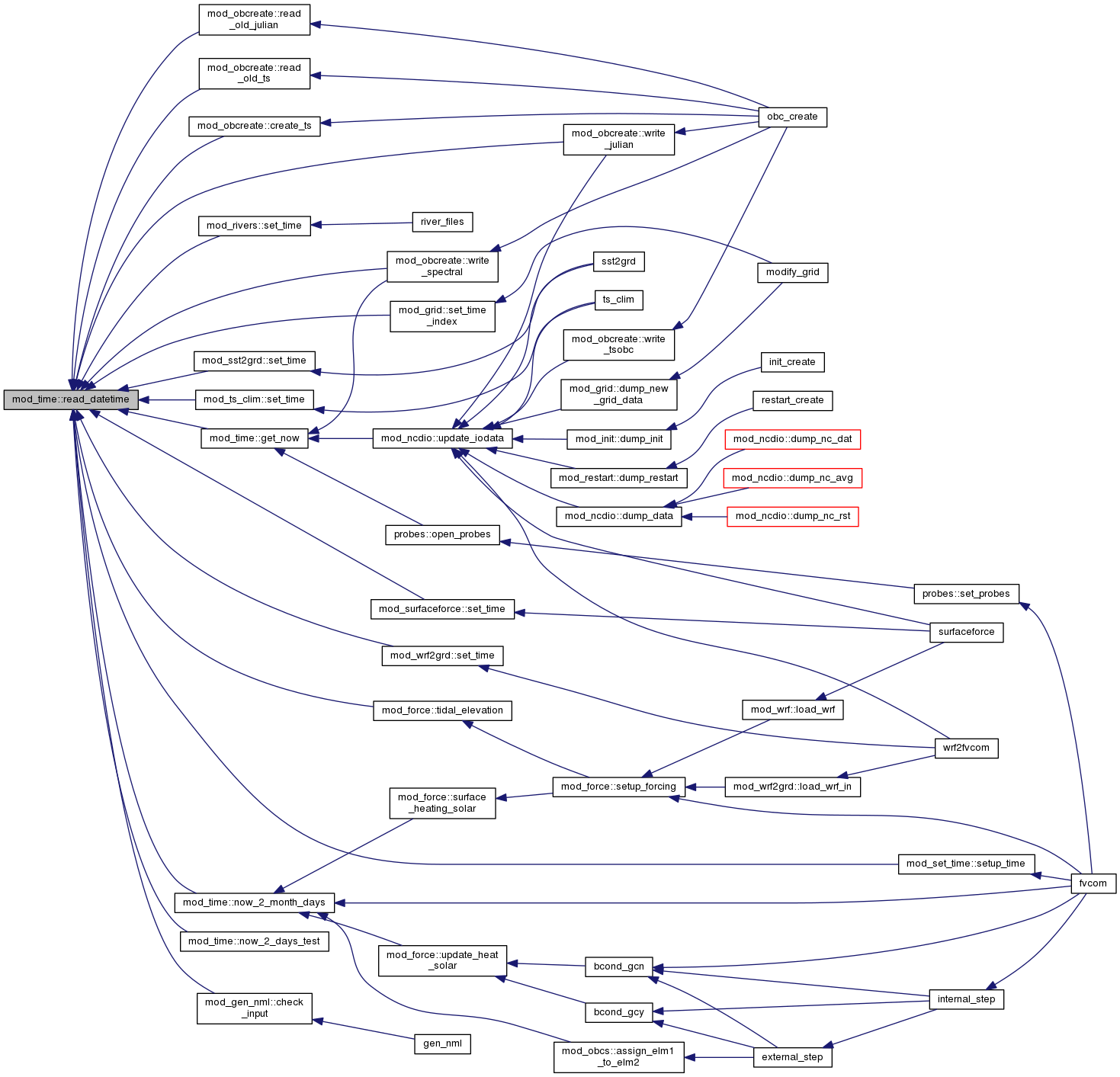
type(time) function mod_time::read_time | ( | character(len=*) | timestr, |
integer | status, | ||
character(len=*), intent(in), optional | TZONE | ||
) |
Here is the call graph for this function:

Here is the caller graph for this function:
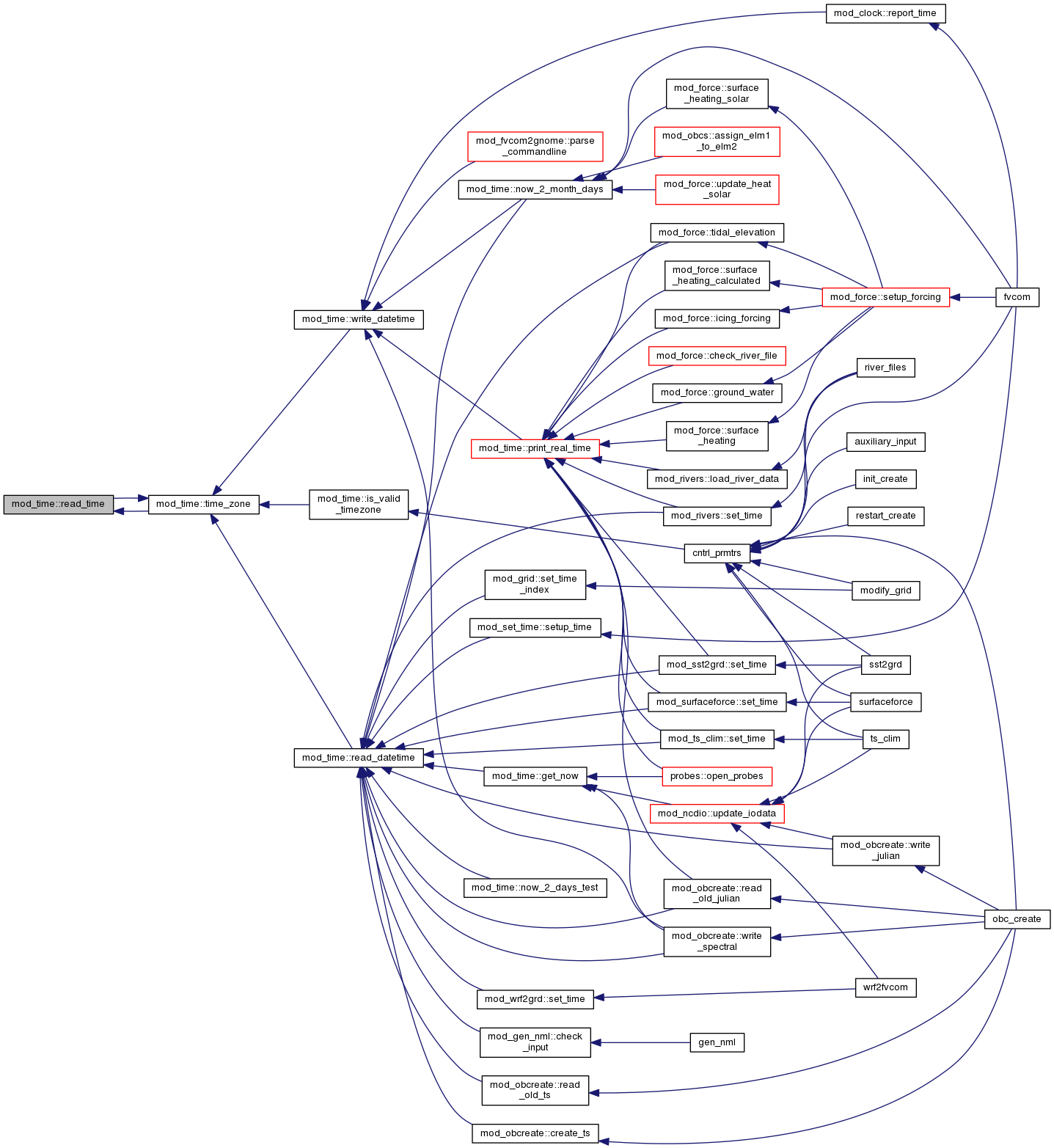
real(dp) function mod_time::seconds | ( | type(time), intent(in) | MJD | ) |
Here is the caller graph for this function:
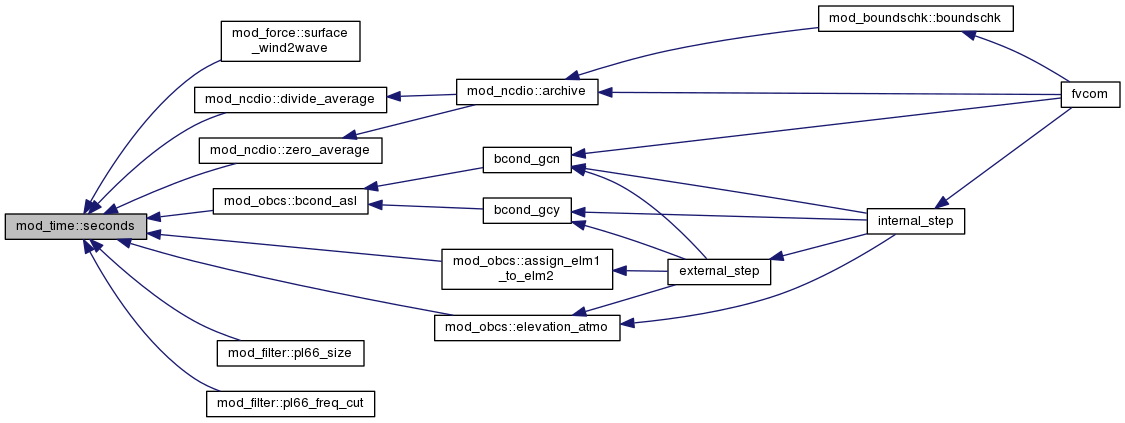
type(time) function mod_time::seconds2time_dbl | ( | real(dp), intent(in) | SECS | ) |
Here is the call graph for this function:

Here is the caller graph for this function:

type(time) function mod_time::seconds2time_flt | ( | real(spa), intent(in) | SECS | ) |
Here is the call graph for this function:

type(time) function mod_time::seconds2time_int | ( | integer, intent(in) | SECS | ) |
Here is the call graph for this function:

Here is the call graph for this function:

type(time) function mod_time::subtract_time | ( | type(time), intent(in) | time1, |
type(time), intent(in) | time2 | ||
) |
Here is the call graph for this function:

type(time) function, dimension(size(time1)) mod_time::subtract_time_1 | ( | type(time), dimension(:), intent(in) | time1, |
type(time), dimension(:), intent(in) | time2 | ||
) |
type(time) function, dimension(size(time2)) mod_time::subtract_time_1a | ( | type(time), intent(in) | time1, |
type(time), dimension(:), intent(in) | time2 | ||
) |
type(time) function, dimension(size(time1,1),size(time1,2)) mod_time::subtract_time_2 | ( | type(time), dimension(:,:), intent(in) | time1, |
type(time), dimension(:,:), intent(in) | time2 | ||
) |
type(time) function, dimension(size(time1,1),size(time1,2)) mod_time::subtract_time_2a | ( | type(time), dimension(:,:), intent(in) | time1, |
type(time), intent(in) | time2 | ||
) |
type(time) function, dimension(size(time1)) mod_time::subtract_time_a1 | ( | type(time), dimension(:), intent(in) | time1, |
type(time), intent(in) | time2 | ||
) |
type(time) function, dimension(size(time2,1),size(time2,2)) mod_time::subtract_time_a2 | ( | type(time), intent(in) | time1, |
type(time), dimension(:,:), intent(in) | time2 | ||
) |
integer function mod_time::time2ncitime | ( | type(time), intent(in) | MJD, |
type(time), intent(in) | RJD, | ||
integer, intent(out) | D, | ||
integer, intent(out) | MS | ||
) |
type(time) function mod_time::time_div_flt | ( | type(time), intent(in) | MJD, |
real(spa), intent(in) | flt | ||
) |
type(time) function mod_time::time_div_long | ( | type(time), intent(in) | MJD, |
integer(itime), intent(in) | long | ||
) |
type(time) recursive function mod_time::time_x_dbl | ( | type(time), intent(in) | MJD, |
real(dp), intent(in) | dbl | ||
) |
Here is the call graph for this function:

Here is the call graph for this function:

type(time) function mod_time::time_x_long | ( | type(time), intent(in) | MJD, |
integer(itime), intent(in) | long | ||
) |
Here is the call graph for this function:

type(time) function mod_time::time_zone | ( | character(len=*), intent(in) | TZONE, |
integer, intent(out) | status | ||
) |
Here is the call graph for this function:

Here is the caller graph for this function:
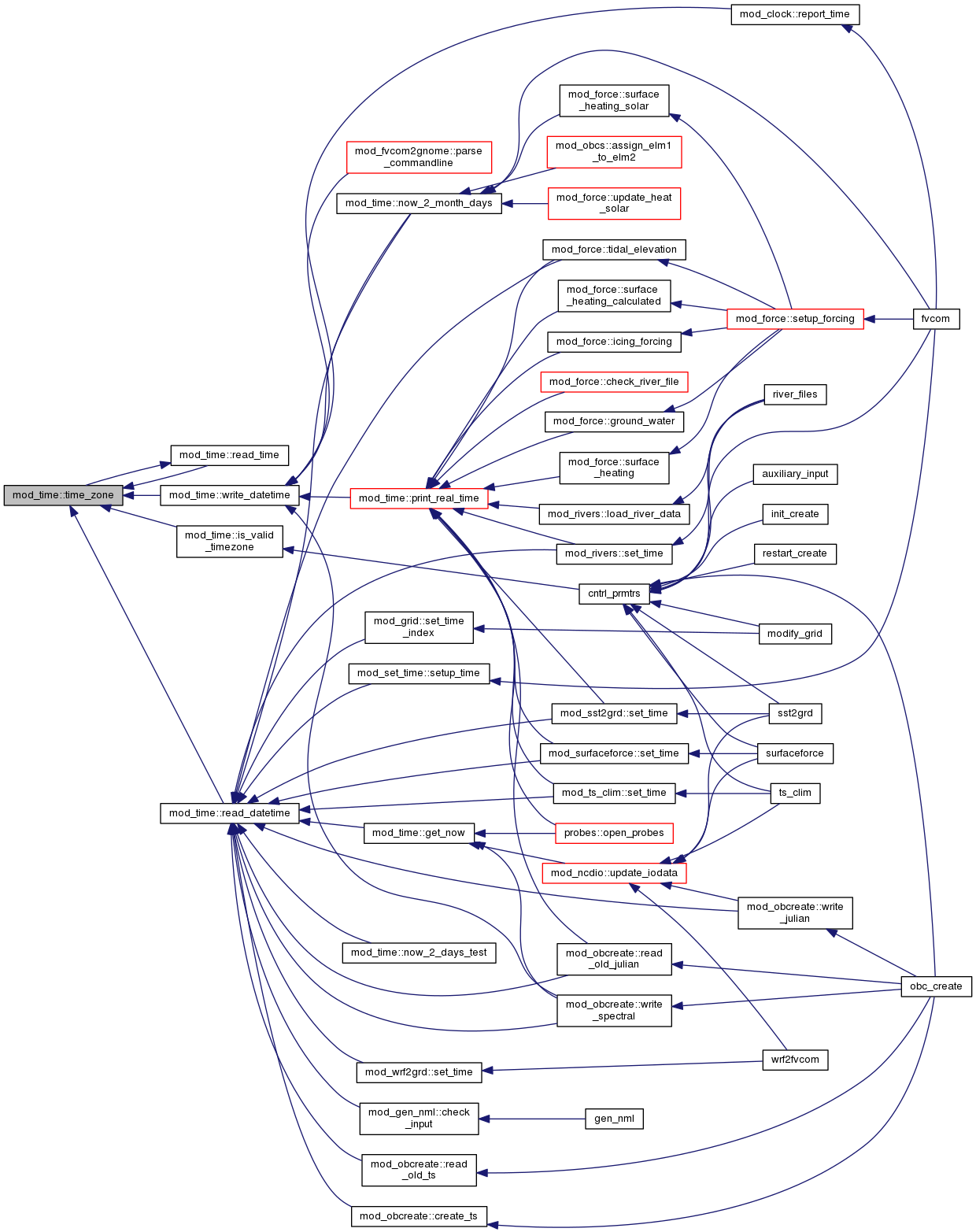
character(len=80) function mod_time::write_datetime | ( | type(time), intent(in) | mjdin, |
integer, intent(in) | prec, | ||
character(len=*), intent(in) | TZONE | ||
) |
Here is the call graph for this function:

Here is the caller graph for this function:
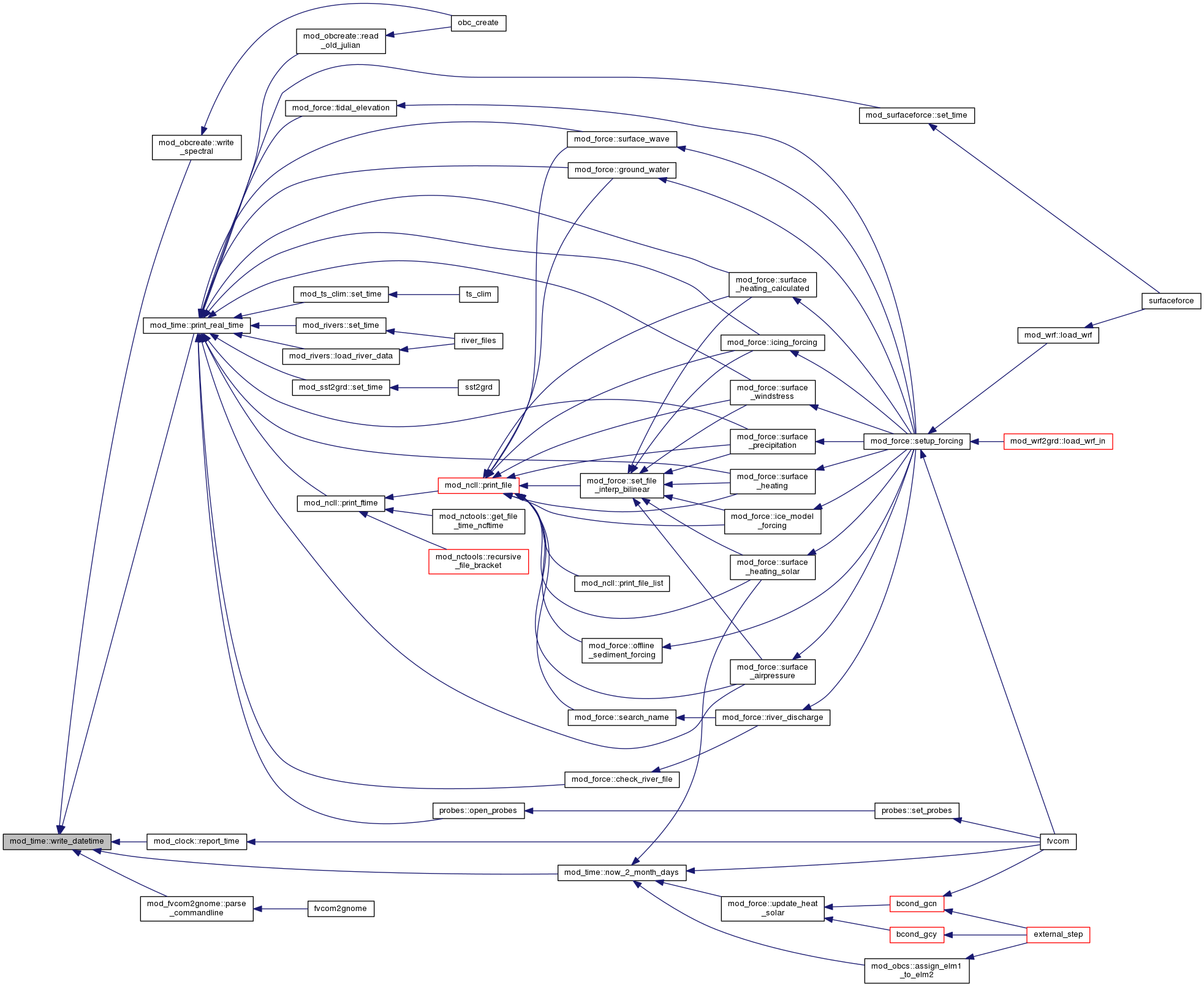
Variable Documentation
integer, parameter mod_time::itime = SELECTED_INT_KIND(18) |
integer(itime), parameter mod_time::million = 10**6 |
integer mod_time::mpi_time |
integer(itime), parameter mod_time::spd = 86400 |